HollowCodable is a codable customization using property wrappers library for Swift.
- Make Complex Codable Serializate a breeze with declarative annotations!
struct YourModel: MappingCodable {
@Immutable
var id: Int
var url: URL?
@Immutable @BoolCoding
var bar: Bool?
@SecondsSince1970DateCoding
var timestamp: Date?
@DateFormatCoding<Hollow.DateFormat.yyyy_mm_dd_hh_mm_ss>
var time: Date?
@ISO8601DateCoding
var iso8601: Date?
@HexColorCoding
var color: HollowColor?
@EnumCoding<TextEnumType>
var type: TextEnumType?
@DecimalNumberCoding
var amount: NSDecimalNumber?
@RGBAColorCoding
var backgroundColor: HollowColor?
var dict: DictAA?
struct DictAA: MappingCodable {
@DecimalNumberCoding
var amount: NSDecimalNumber?
}
static var codingKeys: [ReplaceKeys] {
return [
ReplaceKeys.init(replaceKey: "color", originalKey: "hex_color"),
ReplaceKeys.init(replaceKey: "url", originalKey: "github"),
ReplaceKeys.init(replaceKey: "backgroundColor", originalKey: "background_color"),
]
}
}
- Like this json:
{
"code": 200,
"message": "test case.",
"data": [{
"id": 2,
"title": "Harbeth Framework",
"imageURL": "https://upload-images.jianshu.io/upload_images/1933747-4bc58b5a94713f99.jpeg",
"github": "https://github.com/yangKJ/Harbeth",
"amount": "23.6",
"hex_color": "#FA6D5B",
"type": "text1",
"timestamp" : 590277534,
"bar": 1,
"time": "2024-05-29 23:49:55",
"iso8601": "2023-07-23T23:36:38Z",
"background_color": {
"red": 255,
"green": 128,
"blue": 128
},
"dict": {
"amount": "52.9",
}
}, {
"id": 7,
"title": "Network Framework",
"imageURL": "https://upload-images.jianshu.io/upload_images/1933747-4bc58b5a94713f99.jpeg",
"github": "https://github.com/yangKJ/RxNetworks",
"amount": 120.3,
"hex_color": "#1AC756",
"type": "text2",
"timestamp" : 590288534,
"bar": "yes",
"time": "2024-05-29 20:23:46",
"iso8601": "2023-05-23T09:43:38Z",
"background_color": {
"red": 200,
"green": 63,
"blue": 94
},
"dict": {
"amount": 200,
}
}]
}
- @Immutable: Becomes an immutable property.
- @Base64DataCoding: For a Data property that should be serialized to a Base64 encoded String.
- @BoolCoding: Sometimes an API uses an
Int
orString
for a booleans. - @DateFormatterCoding: Date property that should be serialized using the customized DataFormat.
- @ISO8601DateFormatter: Date property that should be serialized using the ISO8601DateFormatter.
- @Since1970DateCoding: Date property that should be serialized to Since1970.
- @DecimalNumberCoding: Deserialization the
String
、Double
、Float
、CGFloat
、Int
orInt64
to a NSDecimalNumber property. - @EnumCoding: Serialization to enumeration with RawRepresentable.
- @HexColorHasCoding: UIColor/NSColor property that should be serialized to hex string.
- @RGBAColorCoding: RGBA color serialization/deserialization.
- @CustomizedCoding: Customized coding serialization/deserialization.
Booming is a base network library for Swift. Developed for Swift 5, it aims to make use of the latest language features. The framework's ultimate goal is to enable easy networking that makes it easy to write well-maintainable code.
This module is serialize and deserialize the data, Replace HandyJSON.
🎷 Example of use in conjunction with the network part:
func request(_ count: Int) -> Observable<[CodableModel]> {
CodableAPI.cache(count)
.request(callbackQueue: DispatchQueue(label: "request.codable"))
.deserialized(ApiResponse<[CodableModel]>.self, mapping: CodableModel.self)
.compactMap({ $0.data })
.observe(on: MainScheduler.instance)
.catchAndReturn([])
}
CocoaPods is a dependency manager. For usage and installation instructions, visit their website. To integrate using CocoaPods, specify it in your Podfile:
If you wang using Codable:
pod 'HollowCodable'
The general process is almost like this, the Demo is also written in great detail, you can check it out for yourself.🎷
Tip: If you find it helpful, please help me with a star. If you have any questions or needs, you can also issue.
Thanks.🎇
- 🎷 E-mail address: [email protected] 🎷
- 🎸 GitHub address: yangKJ 🎸
Buy me a coffee or support me on GitHub.
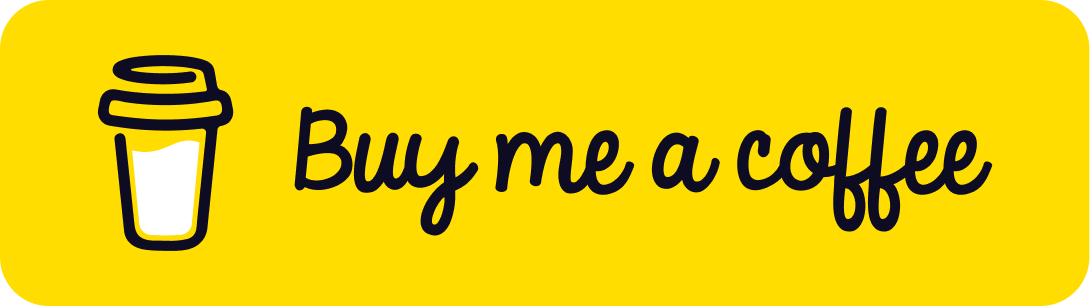
Booming is available under the MIT license. See the LICENSE file for more info.